Keil C compiler provides number of extensions for standarad C function declerations. These extensions allows you to:
- Specify a function as an interrupt procedure
- Choose the register bank used
- Select memory model
►Function Declaration:
[Return_type] Fucntion_name ( [Arguments] ) [Memory_model] [reentrant] [interrupt n] [using n]
Return_type: The type of value returned from the function. If return type of a function is not specified, int is assumed by default.
Function_name: Name of function.
Arguments: Arguments passed to function.
Options:
These are options that you can specify along with function declaration.
Memory_model: explicit memory model (Large, Compact, Small) for the function. Example:
CODE:
int add_number (int a, int b) Large
reentrant: To indicate if the function is reentrant or recursive. This option is explained later in the tutorial.
interrupt: Indicates that function is an interrupt service routine. This option is explained later in the tutorial.
using: Specify register bank to be used during function execution. We have three register banks in 8051 architecture. These register banks are specified using number 0 for Bank 0 to 3 for Bank 3 as shown in example
CODE:
void function_name () using 2{ //function uses Bank 2
//function code
}
//function code
}
►Interrupt Service Routines:
A function can be specified as an interrupt service routine using the keyword interrupt and interrupt number. The interrupt number indicates the interrupt for which the function is declared as service routine.
Following table describes the default interrupts:

As 8051 vendors create new parts, more interrupts are added. Keil C51 compiler supports interrupt functions for 32 interrupts (0-31). Use the interrupt vector address in the following table to determine the interrupt number.

The interrupt function can be declared as follows:
CODE:
void isr_name (void) interrupt 2 {
// Interrupt routine code
}
// Interrupt routine code
}
Please make sure that interrupt service routines should not have any arguments or return type except void.
►Reentrant Functions:
In ANSI C we have recursive function, to meet the same requirement in embedded C, we have reentrant function. These functions can be called recursively and can be called simultaneously by two or more processes.
Now you might be thinking, why special definition for recursive functions?
Well you must know how these functions work when they are called recursively. when a function is running there is some runtime data associated with it, like local variables associated with it etc. when the same function called recursively or two process calls same function, CPU has to maintain the state of function along with its local variables.
Reentrant functions can be defined as follows:
CODE:
void function_name (int argument) reentrant {
//function code
}
//function code
}
Each reentrant function has reentrant stack associated with it, which is defined by startup.A51 file. Reentrant stack area is simulated internal or external memory depending upon the memory model used:
- Small model reentrant functions simulate reentrant stack in idata memory.
- Compant model reentrant functions simulate reentrant stack in pdata memory.
- Large model reentrant functions simulate reentrant stack in xdata memory.
►Real-time Function Tasks:
Keil or C51 provides support for real-time operating system (RTOS) RTX51 Full and RTX51 Tiny. Real-time function task are declared using _task_ and _priority_ keywords. The _task_ defines a function as real-time task. The _priority_ keyword specify the priority of task.
Fucntions are declared as follows:
CODE:
void func (void) _task_ Number _priority_ Priority {
//code
}
//code
}
where:
Number: is task ID from 0 to 255 for RTX51 Full and 0 to 15 for RTX51 Tiny.
Priority: is priority of task.
Real-time task functions must be declared with void return type and void argument list (say no arguments passed to task function).
►Basic of a C program
As we already discussed, Keil C is not much different from a normal C program. If you know assembly, writing a C program is not a problem, only thing you have to keep in mind is forget your controller has general purpose registers, accumulators or whatever. But do not forget about Ports and other on chip peripherals and related registers to them.
In basic C, all programs have atleast one function which is entry point for your application that function is named as "main" function. Similarly in keil, we will have a main function, in which all your application specific work will be defined. Lets move further deep into the working of applications and programs.
When you run your C programs in your PC or computer, you run them as a child program or process to your Operating System so when you exit your programs (exits main function of program) you come back to operating system. Whereas in case of embedded C, you do not have any operating system running in there. So you have to make sure that your program or main file should never exit. This can be done with the help of simple while(1) or for(;;) loop as they are going to run infinitely. Following layout provides a skeleton of Basic C program.
CODE:
void main(){
//Your one time initialization code will come here
while(1){
//while 1 loop
//This loop will have all your application code
//which will run infinitely
}
}
//Your one time initialization code will come here
while(1){
//while 1 loop
//This loop will have all your application code
//which will run infinitely
}
}
When we are working on controller specific code, then we need to add header file for that controller. I am considering you have already gone through "Keil Microvision" tutorial. After project is created, add the C file to project. Now first thing you have to do is adding the header file. All you have to do is right click in editor window, it will show you correct header file for your project.
Figure below shows the windows context for adding header file to your c file.
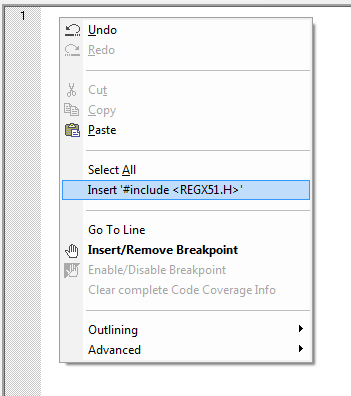
►Writing Hardware specific code
In harware specific code, we use hardware peripherals like ports, timers and uart etc. Do not forget to add header file for controller you are using, otherwise you will not be able to access registers related to peripherals.
Lets write a simple code to Blink LED on Port1, Pin1.
CODE:
#include //header file for 89C51
void main(){
//main function starts
unsigned int i;
//Initializing Port1 pin1
P1_1 = 0; //Make Pin1 o/p
while(1){
//Infinite loop main application
//comes here
for(i=0;i<1000;i++)
; //delay loop
P1_1 = ~P1_1;
//complement Port1.1
//this will blink LED connected on Port1.1
}
}
void main(){
//main function starts
unsigned int i;
//Initializing Port1 pin1
P1_1 = 0; //Make Pin1 o/p
while(1){
//Infinite loop main application
//comes here
for(i=0;i<1000;i++)
; //delay loop
P1_1 = ~P1_1;
//complement Port1.1
//this will blink LED connected on Port1.1
}
}
You can now try out more programs. "Practice makes a man perfect".
In next section of this tutorial, we will learn how to mix C and assembly codes.
►Interfacing C program to Assembler
You can easily interface your programs to routines written in 8051 Assembler. All you need to do is follow few programming rules, you can call assembly routines from C and vice-versa. Public variables declared in assembly modules are available to your C program.
There maybe several reasons to call an assembly routine like faster execution of program, accessing SFRs directly using assembly etc. In this part of tutorial we will discuss how to write assembly progarms that can be directly interfaced with C programs.
For any assembly routine to be called from C program, you must know how to pass parameters or arguements to fucntion and get return values from a function.
►Segment naming
C51 compiler generates objects for every program like program code, program data and constant data. These objects are stored in segments which are units of code or data memory. Segment naming is standard for C51 compiler, so every assembly program need to follow this convention.
Segment names include module_name which is the name of the source file in which the object is declared. Each segment has a prefix that corresponds to memory type used for the segment. Prefix is enclosed in question marks (?). The following is the list of the standard segment name prefixes:
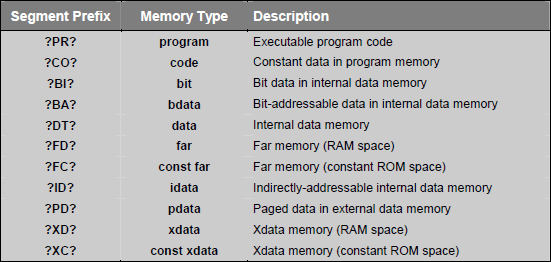
►Data Objects:
Data objects are the variables and constants you declare in your C programs. The C51 compiler generates a saperate segment for each memory type for which variable is declared. The following table lists the segment names generated for different variable data objects.

►Program Objects:
Program onjects includes code generated for C programs functions by C51 compiler. Each function in a source module is assigned a separate code segment using the ?PR?function_name?module_name naming convention. For example, for a function name send_char in file name uart.c will have a segment name of ?PR?SEND_CHAR?UART.
C51 compiler creates saperate segments for local variables that are declared within the body of a function. Segment naming conventions for different memory models are given in following tables:
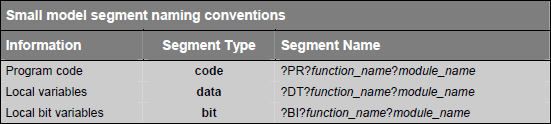

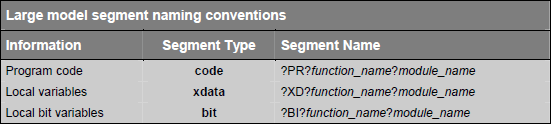
Function names are modified slightly depending on type of function (functions without arguments, functions with arguments and reentrant functions). Following tables explains the segment names:
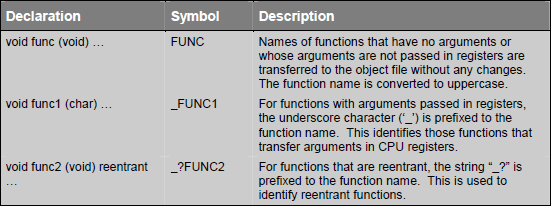
In next section we will learn regarding defining functions which can be called from any C function and how a C function can be called from assembly.
►Function Parameters
C51 make use of registers and memory locations for passing parameters. By default C function pass up to three parameters in registers and further parameters are passed in fixed memory locations. You can disable parameter passing in register using NOREGPARMS keyword. Parameters are passed in fixed memory location if parameter passing in register is disabled or if there are too many parameters to fit in registers.
►Parameter passing in registers
C functions may pass parameter in registers and fixed memory locations. Following table gives an idea how registers are user for parameter passing.
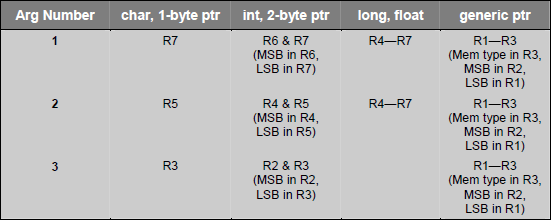
Following example explains a little more clearly the parameter passing technique:

►Parameter passing in Fixed Memory Locations
Parameters passed to assembly routines in fixed memory lcoation use segments named
?function_name?BYTE : All except bit parameters are defined in this segment.
?function_name?BIT : Bit parameters are defined in this segment.
All parameters are assigned in this space even if they are passed using registers. Parameters are stored in the order in which they are declared in each respective segment.
The fixed memory locations used for parameters passing may be in internal data memory or external data memory depending upon the memory model used. The SMALL memory model is the most efficient and uses internal data memory for parameter segment. The COMPACT and LARGE models use external data memory for the parameter passing segments.
►Fucntion Return Values
Function return values are always passed using CPU registers. The following table lists the possible return types and the registers used for each.
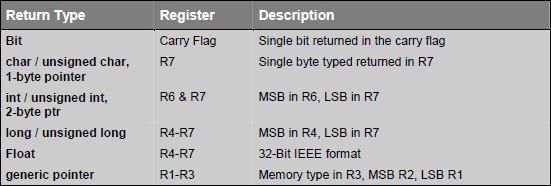
►Example
Following example shows how these segment and function decleration is done in assembler.
CODE:
;Assembly program example which is compatible
;and called from any C program
;lets say asm_test.asm is file name
name asm_test
;We are going to write a function
;add which can be used in c programs as
; unsigned long add(unsigned long, unsigned long);
; as we are passing arguments to function
;so function name is prefixed with '_' (underscore)
;code segment for function "add"
?PR?_add?asm_test segment code
;data segment for function "add"
?DT?_add?asm_test segment data
;let other function use this data space for passing variables
public ?_add?BYTE
;make function public or accessible to everyone
public _add
;define the data segment for function add
rseg ?DT?_add?asm_test
?_add?BYTE:
parm1: DS 4 ;First Parameter
parm2: ds 4 ;Second Parameter
;either you can use parm1 for reading passed value as shown below
;or directly use registers used to pass the value.
rseg ?PR?_add?asm_test
_add:
;reading first argument
mov parm1+3,r7
mov parm1+2,r6
mov parm1+1,r5
mov parm1,r4
;param2 is stored in fixed location given by param2
;now adding two variables
mov a,parm2+3
add a,parm1+3
;after addition of LSB, move it to r7(LSB return register for Long)
mov r7,a
mov a,parm2+2
addc a,parm1+2
;store second LSB
mov r6,a
mov a,parm2+1
addc a,parm1+1
;store second MSB
mov r5,a
mov a,parm2
addc a,parm1
;store MSB of result and return
;keil will automatically store it to
;varable reading the resturn value
mov r4,a
ret
end
;and called from any C program
;lets say asm_test.asm is file name
name asm_test
;We are going to write a function
;add which can be used in c programs as
; unsigned long add(unsigned long, unsigned long);
; as we are passing arguments to function
;so function name is prefixed with '_' (underscore)
;code segment for function "add"
?PR?_add?asm_test segment code
;data segment for function "add"
?DT?_add?asm_test segment data
;let other function use this data space for passing variables
public ?_add?BYTE
;make function public or accessible to everyone
public _add
;define the data segment for function add
rseg ?DT?_add?asm_test
?_add?BYTE:
parm1: DS 4 ;First Parameter
parm2: ds 4 ;Second Parameter
;either you can use parm1 for reading passed value as shown below
;or directly use registers used to pass the value.
rseg ?PR?_add?asm_test
_add:
;reading first argument
mov parm1+3,r7
mov parm1+2,r6
mov parm1+1,r5
mov parm1,r4
;param2 is stored in fixed location given by param2
;now adding two variables
mov a,parm2+3
add a,parm1+3
;after addition of LSB, move it to r7(LSB return register for Long)
mov r7,a
mov a,parm2+2
addc a,parm1+2
;store second LSB
mov r6,a
mov a,parm2+1
addc a,parm1+1
;store second MSB
mov r5,a
mov a,parm2
addc a,parm1
;store MSB of result and return
;keil will automatically store it to
;varable reading the resturn value
mov r4,a
ret
end
Now calling this above function from a C program is very simple. We make function call as normal function as shown below:
CODE:
extern unsigned long add(unsigned long, unsigned long);
void main(){
unsigned long a;
a = add(10,30);
//a will have 40 after execution
while(1);
}
void main(){
unsigned long a;
a = add(10,30);
//a will have 40 after execution
while(1);
}
sưu tầm và biên tập từ nhiều nguồn, mọi ý kiến về kỹ thuật xin vui long liên hệ
soundandlightvn@gmail.com – 0906 715 077 rất mong được đóng góp của các độc giả.
Không có nhận xét nào:
Đăng nhận xét